In today’s digital age, data is everywhere. From social media interactions to online purchases, we are constantly generating vast amounts of data. As a result, the demand for individuals who can analyze and interpret this data has grown exponentially. One of the most powerful tools used for data analysis is Python. With its simple syntax, extensive libraries, and scalability, Python has become the go-to language for data analysts, scientists, and engineers.
Whether you’re a beginner looking to enter the world of data analysis or an experienced programmer seeking to enhance your skills with Python, this comprehensive guide will provide you with all the necessary knowledge to master data analysis with Python. We will cover everything from setting up your python environment to advanced data manipulation and visualization techniques. By the end of this article, you’ll have the skills and understanding to tackle real-world data analysis projects with confidence.
Introduction to Data Analysis and Python
Before diving into the world of data analysis, let’s first understand what it means and why it is important. Data analysis is the process of collecting, cleaning, organizing, and interpreting data to uncover insights and make informed decisions. It involves using various methods and techniques to extract meaningful information from data, such as statistical analysis, data mining, and machine learning.
Python, on the other hand, is a high-level, general-purpose programming language that is widely used in data analysis and scientific computing. It is known for its readability, versatility, and robust libraries such as Pandas, Numpy, and Matplotlib. These libraries provide powerful data structures and tools for data manipulation, analysis, and visualization, making Python a popular choice for data analysis.
Why Use Python for Data Analysis?
With the rise in demand for data-related jobs, there has been a shift towards using Python for data analysis. Here are some reasons why Python is the preferred language for data analysis:
- Ease of Use: Python has a simple and intuitive syntax, making it easy to learn and use for beginners. It also allows you to write code in fewer lines compared to other programming languages, making it more readable and easier to maintain.
- Extensive Libraries: As mentioned earlier, Python has an extensive collection of libraries that provide powerful tools for data analysis. These libraries are continuously updated and maintained by the community, ensuring access to the latest and most efficient methods for data manipulation and analysis.
- Scalability: Python is a high-level language, meaning it is not dependent on hardware or operating systems. This makes it highly scalable, allowing for the processing of large datasets without compromising speed or performance.
Now that we have a basic understanding of data analysis and why Python is the preferred language for it, let’s move on to setting up our Python environment.
Setting Up Your Python Environment
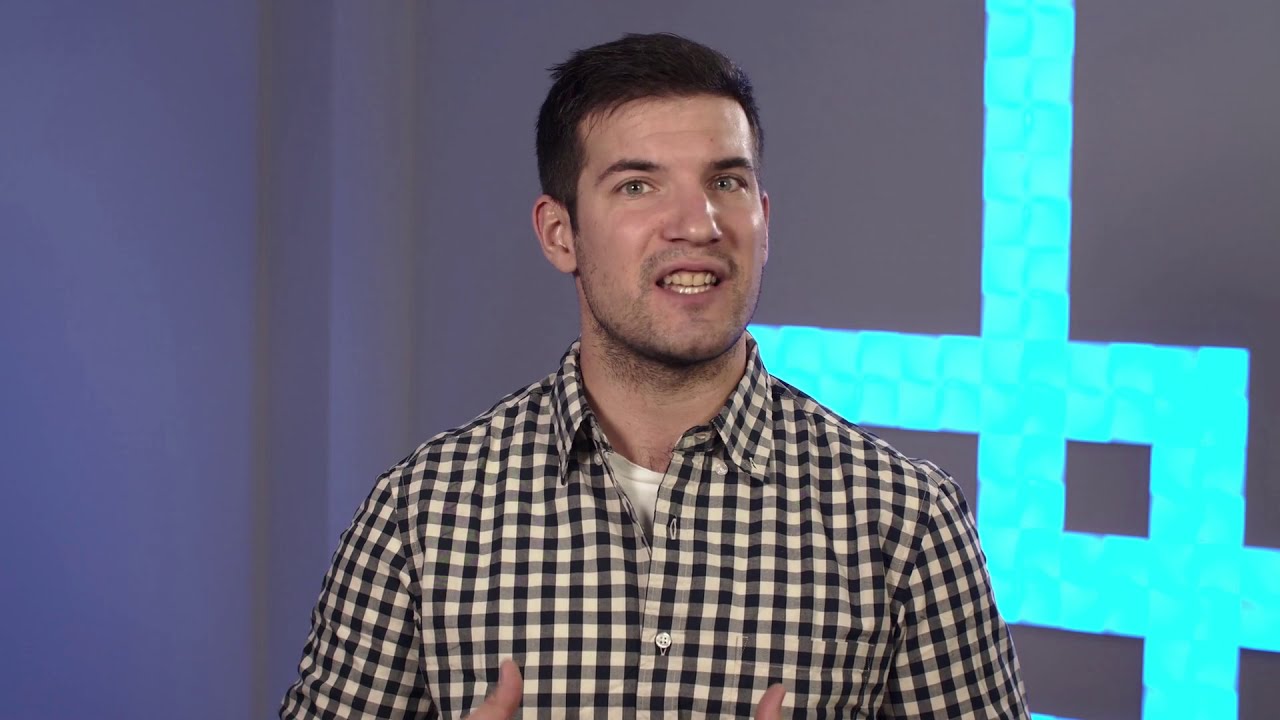
Before we can start analyzing data with Python, we need to set up our environment. This involves installing Python and its necessary dependencies, such as libraries, packages, and an integrated development environment (IDE). In this section, we will cover the steps required to set up your Python environment on Windows, Mac, and Linux.
Installing Python
First, we need to install Python on our system. The following steps demonstrate how to do so on different operating systems:
Windows
- Visit the Python website and click on the “Download” button for the latest version of Python.
- Once the download is complete, run the installer and follow the instructions.
- Make sure to select the option to add Python to your PATH during installation, which will allow you to run Python from any directory on your computer.
- Once the installation is complete, you can verify it by opening the Command Prompt and typing “python –version”. You should see the version number of the Python installed.
Mac
- Visit the Python website and click on the “Download” button for the latest version of Python.
- Once the download is complete, run the installer and follow the instructions.
- Make sure to select the option to add Python to your PATH during installation, which will allow you to run Python from any directory on your computer.
- After the installation is complete, open the Terminal and type “python –version”. You should see the version number of the Python installed.
Linux
Most Linux distributions come with Python pre-installed. However, if you need to install it manually, follow these steps:
- Open the Terminal and type “sudo apt-get update”.
- Type “sudo apt-get install python3.9”, replacing 3.9 with the latest version available.
Installing an IDE
An integrated development environment (IDE) provides a coding environment that makes writing, testing, and debugging code more efficient. There are numerous options available for Python, such as PyCharm, Visual Studio Code, and Jupyter Notebook. For this guide, we will use Jupyter Notebook as it allows us to write and run our code in a browser, making it beginner-friendly.
To install Jupyter Notebook, follow these steps:
- Open the Terminal or Command Prompt and type “pip install jupyter”.
- Once the installation is complete, type “jupyter notebook” to start Jupyter Notebook on your default browser.
Installing Libraries
Now that we have Python and an IDE set up, we need to install the necessary libraries for data analysis. Some essential libraries for data analysis with Python are:
- Pandas: Provides fast, flexible, and expressive data structures for manipulating and analyzing data.
- Numpy: A powerful library for scientific computing and working with multidimensional arrays.
- Matplotlib: A library used for creating visualizations such as line plots, histograms, scatter plots, and more.
- Scipy: A collection of mathematical algorithms and functions for scientific computing.
To install these libraries, open the Terminal or Command Prompt and type:
pip install pandas numpy matplotlib scipy
Data Collection and Importing Data
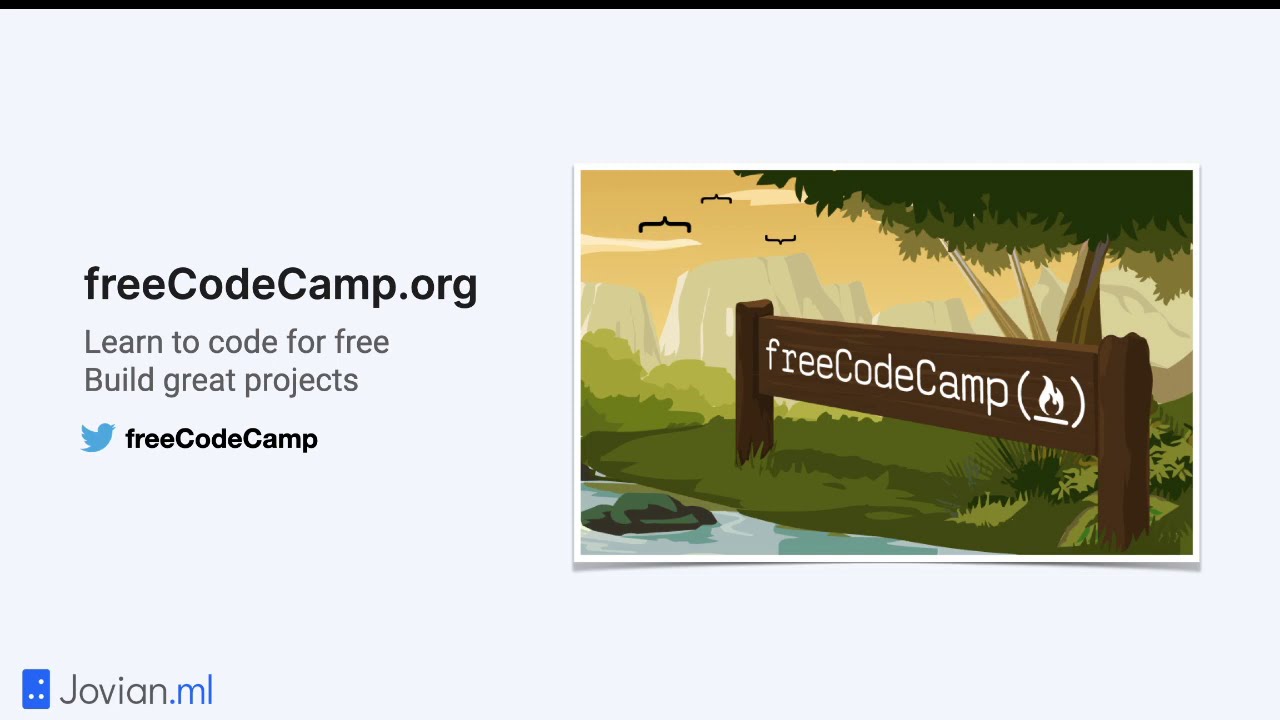
With our environment set up, we can now start working with data. The first step in any data analysis project is to collect and import the data into our environment. There are various sources of data such as CSV files, databases, APIs, web scraping, and more. In this section, we will focus on importing data from a CSV file and a database.
Importing Data from a CSV File
A CSV (Comma Separated Values) file is a common format for storing tabular data. To import a CSV file into our Python environment, we will use the Pandas library.
- First, we need to import the Pandas library into our code using the “import” keyword followed by the library name.
import pandas as pd
- Next, we will use the “read_csv()” function from Pandas to read the CSV file. This function takes in the path of the CSV file and returns a DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types.
data = pd.read_csv('path/to/file.csv')
- We can then view the data by using the “head()” function, which displays the first five rows of the DataFrame.
data.head()
Importing Data from a Database
Another common source of data for data analysts is a database. To connect to a database and import data into our Python environment, we will use the SQLAlchemy library.
- First, we need to import the SQLAlchemy library into our code.
import sqlalchemy
- Next, we need to establish a connection to the database by specifying the database type, username, password, host, and port.
engine = sqlalchemy.create_engine('database-type://username:password@host:port/database-name')
- Once the connection is established, we can use the “read_sql()” function from Pandas to import data from the database into our environment. This function takes in a SQL query and the engine object we created in the previous step.
data = pd.read_sql('SELECT * FROM table_name', engine)
Data Cleaning and Preprocessing
After importing the data, the next step is to clean and preprocess it. Data cleaning involves identifying and correcting any errors or missing values in the dataset, while preprocessing involves transforming the data to make it suitable for analysis. Let’s look at some common data cleaning and preprocessing techniques.
Handling Missing Values
Missing values are common in datasets and can cause issues during analysis. To handle missing values, we have various options such as:
- Dropping Rows: If the number of missing values is small, we can choose to drop those rows using the “dropna()” function from Pandas.
data.dropna()
- Filling with Values: We can also choose to fill the missing values with a specific value, such as the mean or median using the “fillna()” function.
data.fillna(data['column'].mean())
Handling Outliers
Outliers are extreme values that deviate significantly from the rest of the data. To identify and remove outliers, we can use statistical methods such as the Interquartile Range (IQR) and boxplots.
Q1 = data['column'].quantile(0.25)
Q3 = data['column'].quantile(0.75)
IQR = Q3 - Q1
lower_range = Q1 - (1.5 * IQR)
upper_range = Q3 + (1.5 * IQR)
# Remove outliers from the column
data = data[(data['column'] > lower_range) & (data['column'] < upper_range)]
Data Transformation
Data transformation involves converting data from one form to another, making it more suitable for analysis. Some common transformations include:
- Scaling Data: When working with numerical data, we may need to scale it to a specific range to avoid bias in our analysis. We can do this using methods such as Min-Max scaling and Standardization.
from sklearn.preprocessing import MinMaxScaler, StandardScaler
# Min-Max Scaling
scaler = MinMaxScaler()
data['column'] = scaler.fit_transform(data[['column']])
# Standardization
scaler = StandardScaler()
data['column'] = scaler.fit_transform(data[['column']])
- Encoding Categorical Data: Categorical data needs to be converted into numerical data before using it in a machine learning algorithm. We can do this using methods such as Label Encoding and One-Hot Encoding.
from sklearn.preprocessing import LabelEncoder, OneHotEncoder
# Label Encoding
label_encoder = LabelEncoder()
data['column'] = label_encoder.fit_transform(data['column'])
# One-Hot Encoding
onehot_encoder = OneHotEncoder()
data = onehot_encoder.fit_transform(data['column'].values.reshape(-1,1)).toarray()
Exploratory Data Analysis (EDA)
With our data cleaned and preprocessed, we can now move on to exploratory data analysis (EDA). EDA is an essential step in any data analysis project as it allows us to understand the data better and uncover insights that can guide our analysis.
Descriptive Statistics
Descriptive statistics provides a summary of the data, such as mean, median, mode, standard deviation, and quartiles. We can use the “describe()” function from Pandas to get a quick overview of the data.
data.describe()
Data Visualization
Data visualization is a powerful tool that helps us understand the data in a visual format. It allows us to identify patterns, trends, and relationships between variables. Some common plots used for data visualization are:
- Histograms: Used to visualize the distribution of numerical data.
import matplotlib.pyplot as plt
plt.hist(data['column'])
plt.xlabel('Column')
plt.ylabel('Frequency')
plt.title('Distribution of Column')
plt.show()
- Scatter Plots: Used to visualize the relationship between two numerical variables.
plt.scatter(data['column1'], data['column2'])
plt.xlabel('Column 1')
plt.ylabel('Column 2')
plt.title('Scatter Plot')
plt.show()
- Bar Charts: Used to compare categorical data.
plt.bar(data['column1'], data['column2'])
plt.xlabel('Column 1')
plt.ylabel('Column 2')
plt.title('Bar Chart')
plt.show()
Advanced Data Manipulation with Pandas
Pandas provides a wide range of functions and methods for manipulating data. In this section, we will cover some advanced techniques for data manipulation using Pandas.
Grouping Data
Grouping data involves splitting the data into groups based on a specific variable and performing operations on each group. For example, we can group a dataset by a certain country and calculate the average income for each country.
grouped_data = data.groupby('country')['income'].mean()
Joining and Merging Data
In some cases, we may need to combine two or more datasets to perform analysis. We can do this using the “merge()” function in Pandas.
merged_data = pd.merge(data1, data2, on='column')
Reshaping Data
We may also need to reshape our data to make it suitable for analysis. Pandas provides functions such as “pivot()” and “melt()” for reshaping data.
# Pivot
pivoted_data = data.pivot(index='column1', columns='column2', values='value')
# Melt
melted_data = pd.melt(data, id_vars=['column1', 'column2'], value_vars=['value1', 'value2'])
Statistical Analysis with Python
Python has a rich collection of libraries for performing statistical analysis on data. Some of these libraries include Scipy, Statsmodels, and Scikit-learn. In this section, we will cover some basic statistical techniques using the Scipy library.
Hypothesis Testing
Hypothesis testing is used to determine whether the results of an experiment are statistically significant or occurred by chance. We can perform hypothesis testing using the “ttest_ind()” function from Scipy.
from scipy.stats import ttest_ind
# Perform t-test between two groups of data
t_stat, p_value = ttest_ind(group1_data, group2_data)
Correlation Analysis
Correlation analysis is used to measure the strength and direction of the relationship between two variables. We can use the “pearsonr()” function from Scipy to calculate the correlation coefficient and p-value.
from scipy.stats import pearsonr
# Calculate correlation coefficient and p-value
corr_coef, p_value = pearsonr(data['variable1'], data['variable2'])
Data Visualization Techniques
As mentioned earlier, data visualization is crucial for understanding and communicating insights from data. In this section, we will cover some advanced data visualization techniques using the Matplotlib library.
Subplots
Subplots allow us to create multiple plots on a single figure, making it easier to compare and analyze data. We can use the “subplot()” function to specify the number of rows, columns, and plot position for each subplot.
plt.subplot(2, 3, 1)
plt.plot(data['column1'])
plt.subplot(2, 3, 2)
plt.scatter(data['column1'], data['column2'])
Heatmaps
Heatmaps are a type of graphical representation that uses colors to visualize data. They are commonly used to represent correlations, matrices, and geographical data. We can create a heatmap using the “imshow()” function from Matplotlib.
import numpy as np
# Create random matrix
matrix = np.random.rand(10, 10)
# Create heatmap
plt.imshow(matrix, cmap='Blues')
plt.colorbar()
plt.show()
Interactive Visualizations with Plotly
Plotly is a Python library that allows us to create interactive visualizations such as bar charts, line graphs, and scatter plots. These interactive plots can be manipulated with various tools such as zoom, hover, and click, making it easier to explore and analyze data.
import plotly.express as px
# Create scatter plot
fig = px.scatter(data, x='column1', y='column2', color='column3', hover_data=['column4'])
fig.show()
Machine Learning Basics
Machine learning involves the use of algorithms to identify patterns and make predictions from data. Python provides powerful libraries such as Scikit-learn and Tensorflow for machine learning tasks. In this section, we will cover some basic machine learning concepts using Scikit-learn.
Data Preparation
Before we can apply machine learning algorithms to our data, we need to prepare it in a specific format. This involves splitting the data into training and test sets, scaling numerical data, and encoding categorical data.
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Scale numerical data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Encode categorical data
from sklearn.preprocessing import OneHotEncoder
onehot_encoder = OneHotEncoder()
y_train = onehot_encoder.fit_transform(y_train.values.reshape(-1,1))
Model Training and Evaluation
Once the data is prepared, we can train our machine learning model using the “fit()” function and evaluate it using the “score()” function.
from sklearn.linear_model import LinearRegression
# Train model
model = LinearRegression()
model.fit(X_train, y_train)
# Evaluate model
print(model.score(X_test, y_test))
Real-world Data Analysis Projects
The best way to master data analysis with Python is by working on real-world projects. In this section, we will cover some project ideas that you can work on to apply your skills and enhance yourknowledge.
Predictive Analysis on Housing Prices
Description: In this project, you can use a dataset containing information about housing prices, such as location, size, number of bedrooms, and other relevant factors. Your task is to build a predictive model that can estimate the price of a house based on its features.
Approach:
- Data Collection: Obtain a dataset containing housing information.
- Data Preprocessing: Clean and preprocess the data, handling missing values and encoding categorical variables.
- Exploratory Data Analysis: Explore the data using visualizations to understand the relationships between features and the target variable (price).
Customer Segmentation for Marketing Strategies
Description: Utilize clustering algorithms to segment customers based on their purchasing behavior. By grouping similar customers together, businesses can tailor marketing strategies more effectively.
Approach:
- Data Collection: Gather data on customer transactions and demographics.
- Data Preprocessing: Prepare the data by scaling numerical features and encoding categorical variables.
- Clustering Analysis: Apply clustering algorithms such as K-means or DBSCAN to segment customers into distinct groups based on their behavior.
Sentiment Analysis on Social Media Posts
Description: Analyze sentiments expressed in social media posts to understand public opinion on a particular topic, product, or event. This can help businesses gauge customer satisfaction and identify areas for improvement.
Approach:
- Data Collection: Scrape social media platforms (e.g., Twitter, Reddit) for posts related to the target topic.
- Text Preprocessing: Clean and preprocess text data by removing stopwords, punctuation, and special characters.
- Sentiment Analysis: Use natural language processing tools to analyze the sentiment of each post (positive, negative, neutral).
Conclusion
In conclusion, mastering data analysis with Python opens up a world of opportunities for extracting valuable insights from data. By following the structured approach outlined in this guide—setting up your environment, collecting and preprocessing data, performing exploratory data analysis, advanced manipulation, statistical analysis, data visualization, and machine learning—you can develop proficiency in leveraging Python for data-driven decision-making.
Remember, practice makes perfect. Working on real-world projects and continuously honing your skills will not only enhance your expertise but also pave the way for exciting career opportunities in data analysis, artificial intelligence, and machine learning fields. Embrace the power of Python for data analysis, and embark on a rewarding journey of discovery and innovation in the realm of data science.