In today’s fast-paced software development landscape, maintaining code quality is of paramount importance. As projects grow in complexity and the demand for speed-to-market increases, developers are tasked with the challenge of writing clean, maintainable, and efficient code. This is where code linters come into play, serving as powerful tools to help developers identify and address code-related issues before they escalate into larger problems.
Introduction to Code Linters: Definition and Purpose
A code linter is a software tool that analyzes source code to identify potential issues, such as syntax errors, stylistic inconsistencies, and violations of coding standards. By providing real-time feedback and highlighting these problems, linters help developers write more robust, reliable, and maintainable code.
Understanding the Importance of Code Linters
Code linters play a crucial role in ensuring the overall quality and consistency of a codebase. By catching errors and enforcing best practices, they help developers:
- Improve Code Readability: Linters can enforce consistent code formatting, naming conventions, and other stylistic guidelines, making the codebase more readable and easier to navigate.
- Catch Potential Bugs: Linters can detect common programming mistakes, such as undefined variables, incorrect type conversions, and logical errors, allowing developers to address these issues early in the development process.
- Enforce Coding Standards: Linters can be configured to enforce a project’s or organization’s specific coding standards, promoting a consistent and maintainable codebase.
- Enhance Collaboration: When integrated into a development workflow, linters can help ensure that all team members are writing code according to the same set of rules, improving collaboration and reducing friction.
- Improve Code Security: Linters can identify potential security vulnerabilities, such as improper input validation or the use of insecure coding practices, helping to mitigate the risk of security breaches.
Exploring the Diversity of Code Linters
Code linters come in various forms, catering to different programming languages, frameworks, and development environments. Some of the most popular and widely used code linters include:
- ESLint: A popular linter for JavaScript and TypeScript, ESLint helps developers identify and fix problematic patterns in their code.
- Pylint: A comprehensive linter for Python, Pylint checks for errors, enforces coding standards, and provides suggestions for improving code quality.
- Rubocop: A linter for Ruby, Rubocop ensures that the codebase adheres to the community-driven Ruby Style Guide.
- Checkstyle: A linter for Java, Checkstyle enforces coding conventions and identifies potential problems in Java code.
- Flake8: A Python linter that combines the functionality of multiple tools, including PyFlakes, pycodestyle, and McCabe complexity checker.
These are just a few examples of the many code linters available, each with its own unique features, strengths, and target programming languages.
Importance of Code Quality in Software Development
In the fast-paced world of software development, maintaining a high level of code quality is essential for the long-term success and sustainability of any project. Let’s explore the key reasons why code quality is so important.
The Consequences of Poor Code Quality
When code quality is neglected, the consequences can be severe and far-reaching. Some of the most common issues associated with poor code quality include:
- Increased Maintenance Costs: Poorly written, buggy, or inconsistent code can make it significantly more difficult and time-consuming to maintain and update the codebase over time.
- Reduced Developer Productivity: Dealing with technical debt and fixing issues in a poorly organized codebase can slow down development and hinder a team’s ability to deliver new features and functionalities.
- Compromised User Experience: Bugs, performance issues, and other problems stemming from poor code quality can directly impact the user experience, leading to frustrated customers and potential loss of business.
- Security Vulnerabilities: Inadequate code quality can introduce security loopholes, making the application vulnerable to cyber attacks and data breaches.
- Diminished Team Morale: Working with a codebase that is difficult to understand and maintain can be demoralizing for developers, leading to decreased job satisfaction and increased turnover.
The Benefits of Maintaining High Code Quality
Investing in maintaining high code quality can bring numerous benefits to software development projects, including:
- Improved Productivity: Well-structured, easily understandable code enables developers to work more efficiently, reducing the time and effort required to implement new features or fix issues.
- Enhanced Scalability: A clean, maintainable codebase makes it easier to adapt and scale the application as the project grows in complexity or user base.
- Reduced Technical Debt: Addressing code quality issues early on helps to minimize the accumulation of technical debt, which can otherwise become a significant burden over time.
- Increased Code Reusability: Consistent, modular code design facilitates the reuse of code components, improving development speed and reducing duplication.
- Improved Code Reliability: Linters and other quality assurance tools help to catch bugs and vulnerabilities before they make it into production, leading to more reliable and stable software.
- Better Collaboration: A shared understanding of coding standards and best practices, enforced by linters, promotes collaboration and reduces friction within development teams.
By prioritizing code quality and leveraging the power of code linters, software development teams can create more robust, efficient, and maintainable applications that deliver a superior user experience.
How Code Linters Work: An Overview
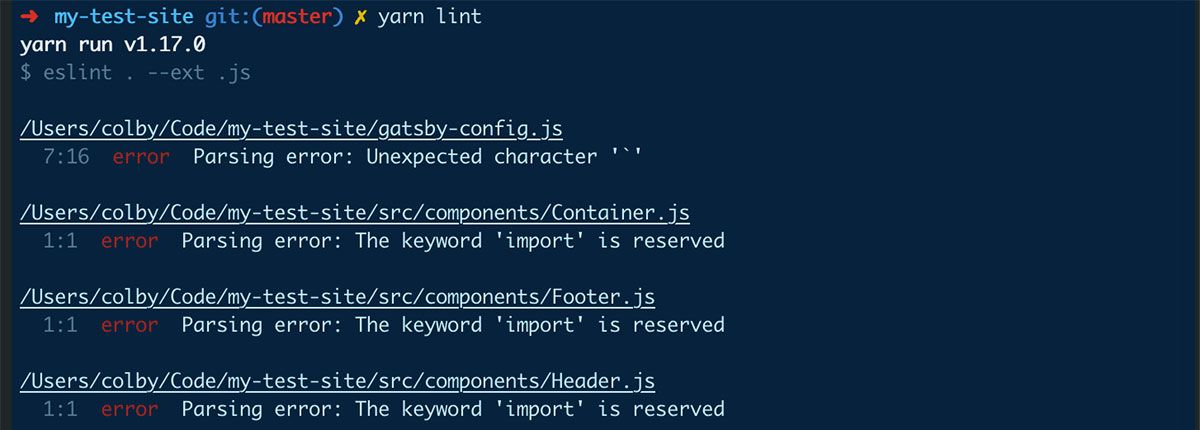
Code linters are powerful tools that analyze source code to identify and report on various types of issues, from syntax errors to stylistic inconsistencies. Understanding the underlying mechanisms of how code linters work can help developers effectively leverage these tools in their development workflows.
The Linting Process
The linting process typically involves the following key steps:
- Lexical Analysis: The linter first breaks down the source code into a series of tokens, such as keywords, identifiers, and operators, using a lexical analyzer.
- Parsing: The linter then uses a parser to construct an abstract syntax tree (AST) from the tokenized code. The AST represents the structure and semantics of the code.
- Rule Evaluation: The linter applies a set of predefined rules to the AST, checking for various types of issues, such as syntax errors, code style violations, and potential runtime problems.
- Issue Reporting: Finally, the linter generates a report, highlighting the identified issues and providing information about their location, severity, and potential remediation strategies.
Types of Code Linting Rules
Code linters typically enforce a wide range of rules to ensure code quality and consistency. These rules can be categorized as follows:
- Syntax Rules: These rules check for proper syntax, such as correct usage of language constructs, proper variable declarations, and appropriate use of control structures.
- Semantic Rules: These rules analyze the meaning and behavior of the code, identifying potential runtime issues like undefined variables, type mismatches, and logical errors.
- Style Rules: Style rules enforce code formatting, naming conventions, and other stylistic guidelines to improve code readability and maintainability.
- Best Practices Rules: These rules promote the use of recommended coding practices, such as proper error handling, efficient resource management, and the avoidance of anti-patterns.
- Security Rules: Security-focused rules identify potential security vulnerabilities, such as improper input validation, the use of insecure APIs, and the presence of known security flaws.
Configuring Linting Rules
Most code linters allow developers to customize the set of rules to be applied, enabling them to tailor the linting process to the specific needs of their project or organization. This configuration can be done through various means, such as:
- Configuration Files: Linters often provide the ability to specify rules and settings in dedicated configuration files, such as
.eslintrc.json
for ESLint orpyproject.toml
for Flake8. - Command-line Options: Many linters support the use of command-line arguments to enable or disable specific rules, adjust rule severity, and fine-tune the linting process.
- IDE Integrations: Linters can be integrated into popular IDEs (Integrated Development Environments), allowing developers to configure and apply linting rules directly within their development environment.
By understanding the underlying mechanisms of code linters and the types of rules they enforce, developers can effectively leverage these tools to improve the quality and consistency of their codebase.
Popular Code Linters: A Comparative Analysis
In the world of software development, there is a wide array of code linters available, each with its own unique features, strengths, and target programming languages. In this section, we will explore and compare some of the most popular code linters to help you determine the best fit for your development needs.
ESLint: The Powerhouse for JavaScript and TypeScript
ESLint is a widely used and highly extensible linter for JavaScript and TypeScript. It is known for its robust rule set, extensive plugin ecosystem, and seamless integration with various development environments.
Key Features:
- Comprehensive set of built-in rules for identifying common JavaScript/TypeScript issues
- Supports ES6, JSX, and TypeScript syntax
- Extensive plugin ecosystem for customizing and extending the rule set
- Integrates well with popular IDEs, such as Visual Studio Code, IntelliJ, and Sublime Text
- Provides detailed and actionable error reports, making it easy to address identified issues
Strengths:
- Unparalleled support for modern JavaScript and TypeScript features
- Highly customizable and configurable to fit the needs of any project
- Strong community and active development, ensuring continuous improvements
Limitations:
- Primarily focused on JavaScript and TypeScript, with limited support for other programming languages
- Steeper learning curve compared to some other linters, due to the vast number of configuration options
Pylint: The Comprehensive Linter for Python
Pylint is a robust linter for the Python programming language, known for its extensive rule set and deep analysis of Python code.
Key Features:
- Comprehensive set of built-in rules for identifying code style, syntax, and logical issues in Python
- Supports Python 2 and 3, as well as various Python frameworks and libraries
- Provides detailed reports with suggestions for improving code quality
- Integrates with popular Python IDEs, such as PyCharm and Visual Studio Code
- Allows for the customization of rule sets and the creation of user-defined rules
Strengths:
- Thorough analysis of Python code, catching a wide range of potential issues
- Highly configurable, enabling developers to tailor the linting process to their specific needs
- Actively maintained and supported by the Python community
Limitations:
- Can be resource-intensive, especially on large codebases, due to its comprehensive analysis
- The learning curve can be steeper compared to some other Python-focused linters
Rubocop: The Code Style Enforcer for Ruby
Rubocop is a widely adopted linter for the Ruby programming language, primarily focused on ensuring code style and adherence to community-driven best practices.
Key Features:
- Enforces the Ruby Style Guide, a set of community-endorsed coding conventions
- Supports a wide range of Ruby versions, including legacy versions
- Provides configurable rule sets and the ability to create custom rules
- Integrates with popular Ruby IDEs, such as RubyMine and Visual Studio Code
- Generates detailed reports with suggested corrections for identified issues
Strengths:
- Ensures consistent code style across Ruby projects, promoting maintainability
- Extensive set of built-in rules based on the Ruby Style Guide
- Strong community support and active development
Limitations:
- Primarily focused on code style, with less emphasis on identifying logical or semantic issues
- May require more configuration to align with a project’s specific coding standards
Checkstyle: The Java Code Quality Guardian
Checkstyle is a widely used linter for the Java programming language, renowned for its comprehensive rule set and focus on code quality.
Key Features:
- Extensive built-in rules for identifying code style, formatting, and potential issues in Java
- Supports various Java coding standards, including Google, Sun, and the Java Community Process (JCP)
- Provides detailed reports with clear explanations and suggestions for improvements
- Integrates with popular Java IDEs, such as IntelliJ IDEA and Eclipse
- Allows for the creation and customization of custom rules
Strengths:
- Comprehensive rule set that covers a wide range of Java code quality aspects
- Supports multiple coding standards, making it adaptable to different projects
- Robust and well-established, with a strong community and active development
Limitations:
- Primarily focused on Java, with limited support for other programming languages
- The configuration and customization process can be more complex compared to some other linters
Flake8: The Versatile Python Linter
Flake8 is a popular Python linter that combines the functionality of several tools, including PyFlakes, pycodestyle, and McCabe complexity checker.
Key Features:
- Integrates the capabilities of multiple Python code quality tools into a single linter
- Checks for syntax errors, style issues, and code complexity problems
- Supports a wide range of Python versions, from 2.7 to the latest 3.x releases
- Provides configurable rule sets and the ability to extend with custom plugins
- Integrates well with various Python IDEs and development environments
Strengths:
- Comprehensive linting capabilities by combining multiple tools
- Streamlined setup and configuration, with sensible default settings
- Actively maintained and supported by the Python community
Limitations:
- While versatile, it may not provide the same level of in-depth analysis as specialized linters like Pylint
- The rule set can be less customizable compared to some other Python linters
By understanding the key features, strengths, and limitations of these popular code linters, you can make an informed decision on which tool(s) best fit your development needs and the specific requirements of your project.
Setting Up a Code Linter in Your Development Environment
Integrating a code linter into your development workflow is a crucial step in maintaining code quality and consistency. In this section, we’ll guide you through the process of setting up a code linter in your development environment, using ESLint as an example.
Installing ESLint
The first step in setting up ESLint is to install it in your project. You can do this using your preferred package manager, such as npm or yarn. Open your terminal or command prompt and run the following command:
npm install --save-dev eslint
This will install the ESLint package as a development dependency in your project.
Configuring ESLint
After installing ESLint, you’ll need to configure it to suit your project’s needs. ESLint provides a variety of configuration options, allowing you to customize the set of rules to be applied and other linting settings.
To create a default ESLint configuration file, you can run the following command:
npx eslint --init
This will guide you through a series of questions, such as the type of project you’re working on, the JavaScript version you’re using, and the desired linting style. Based on your answers, ESLint will generate a configuration file (typically .eslintrc.json
) in your project’s root directory.
Alternatively, you can manually create the .eslintrc.json
file and customize the configuration settings. Here’s an example of a basic ESLint configuration:
{
"env": {
"browser": true,
"es2021": true
},
"extends": "eslint:recommended",
"parserOptions": {
"ecmaVersion": 12,
"sourceType": "module"
},
"rules": {
"indent": ["error", 2],
"linebreak-style": ["error", "unix"],
"quotes": ["error", "double"],
"semi": ["error", "always"]
}
}
This configuration sets up the environment, extends the recommended ESLint rules, and defines a few custom rules for code indentation, line break style, quotes, and semicolon usage.
Integrating ESLint into Your Development Workflow
Once you have the ESLint configuration set up, you can integrate it into your development workflow in various ways:
- IDE Integration: Most popular IDEs, such as Visual Studio Code, IntelliJ IDEA, and Sublime Text, have ESLint integration plugins. These plugins automatically apply linting rules as you write code, providing immediate feedback on any issues.
- Command-line Usage: You can run ESLint from the command line by using the
eslint
command. For example, to lint all JavaScript files in your project, you can use the following command:
npx eslint .
This will scan your entire project and report any identified issues.
- Pre-commit Hooks: To ensure that code changes always adhere to your linting rules, you can set up a pre-commit hook that runs ESLint before allowing a commit to be made. This can be done using tools like Husky or lint-stage.
- Continuous Integration: You can incorporate ESLint into your continuous integration (CI) pipeline to automatically check code quality before merging changes into the main branch. Services like Jenkins, GitLab CI/CD, or GitHub Actions can be configured to run ESLint as part of the build process.
By following these steps, you can effectively set up ESLint in your development environment and leverage its capabilities to improve code quality and maintain consistency across your projects.
Best Practices for Using Code Linters Effectively
While code linters offer valuable assistance in maintaining code quality, maximizing their effectiveness requires adhering to best practices. In this section, we’ll explore some strategies to use code linters efficiently and enhance their impact on your development processes.
Define Coding Standards and Rules
One of the fundamental aspects of leveraging a code linter effectively is establishing clear coding standards and rules for your project. By defining a consistent set of guidelines regarding code style, formatting, and best practices, you provide a foundation for the linter to enforce these standards.
Tip: Create a shared document or style guide that outlines the coding standards to be followed, including naming conventions, indentation preferences, commenting guidelines, and more.
Regularly Review and Update Rules
As coding standards and best practices evolve over time, it’s essential to review and update the rules implemented by your code linter. Periodically revisiting and refining the rule set ensures that your linter remains aligned with the latest industry standards and tailored to the specific requirements of your project.
Tip: Schedule routine reviews of your code linter configuration to identify outdated rules or opportunities for enhancement based on changing coding practices.
Address Issues Promptly
When a code linter highlights issues in your codebase, address them promptly to maintain code quality and consistency. Resolving linting errors and warnings as soon as they are detected prevents the accumulation of technical debt and promotes a healthier codebase overall.
Tip: Prioritize fixing linting issues during code reviews or as part of your regular development workflow to prevent them from escalating into larger problems.
Customize Rules Carefully
While most code linters come with predefined rule sets, you may need to customize certain rules to better suit your project’s unique requirements. When modifying rules or introducing new ones, do so judiciously to avoid creating overly restrictive or counterproductive guidelines.
Tip: Test custom rule configurations on smaller sections of code before applying them globally to assess their impact and ensure they align with your project goals.
Leverage Automation and Integration
Integrating code linters into your development tools and workflows automates the process of code quality assurance, making it easier to enforce coding standards consistently. Automating code linting checks through pre-commit hooks, CI/CD pipelines, and IDE plugins streamlines the feedback loop and enhances productivity.
Tip: Explore available integrations and tools that facilitate seamless incorporation of code linters into your existing development ecosystem, optimizing their utility and accessibility.
By implementing these best practices, you can harness the full potential of code linters to uphold code quality, streamline development processes, and foster collaboration among team members.
Common Issues Detected by Code Linters and How to Resolve Them
Code linters play a crucial role in identifying various issues within codebases, ranging from minor stylistic inconsistencies to potential logic errors. Understanding common problems flagged by code linters and knowing how to address them is essential for maintaining code quality and adherence to coding standards. Let’s explore some typical issues detected by linters and ways to resolve them effectively.
Undefined Variables
One common issue flagged by code linters in many programming languages is the use of variables that have not been defined or initialized. This can lead to runtime errors and unexpected behavior in the code.
Resolution: To fix undefined variable errors, ensure that all variables are properly declared before being used. Check for typos in variable names and verify that variables are assigned values before being accessed.
Unused Imports or Variables
Linters often highlight unused imports or variables in code, indicating unnecessary or redundant declarations that can clutter the codebase and increase complexity.
Resolution: Remove any unused imports or variables from your code to declutter and improve readability. Regularly review and optimize your import statements and variable declarations to eliminate redundancy.
Code Formatting Inconsistencies
Inconsistent code formatting, such as varying indentation levels, mismatched brace styles, or irregular spacing, can make the code harder to read and maintain.
Resolution: Use automated formatting tools or IDE features to enforce consistent code styling throughout your project. Configure your linter to flag formatting inconsistencies and adhere to a unified coding style guide.
Missing Documentation
Lack of documentation or comments within the code can hinder understanding and maintenance efforts, making it challenging for developers to grasp the purpose and functionality of different code segments.
Resolution: Document functions, classes, and complex algorithms with descriptive comments to clarify their intended behavior. Follow a consistent commenting style and ensure that code changes are accompanied by relevant updates to documentation.
Logic Errors and Potential Bugs
While primarily focused on code style, linters may also detect logical errors or potential bugs in the code, such as unreachable code paths, ineffective conditional statements, or unintended variable assignments.
Resolution: Review linter warnings related to logic errors carefully and conduct thorough testing to identify and rectify potential bugs. Refactor code segments that exhibit suspicious behavior or unclear logic to enhance code reliability.
By proactively addressing these common issues identified by code linters, you can enhance code quality, reduce the likelihood of errors, and foster a more maintainable and robust codebase.
Integrating Code Linters into CI/CD Pipelines
Incorporating code linters into your continuous integration and continuous deployment (CI/CD) pipelines offers a systematic approach to enhancing code quality, ensuring consistency, and automating the enforcement of coding standards throughout the software development lifecycle. This section explores the benefits of integrating code linters into CI/CD processes and provides guidance on implementing this practice effectively.
Benefits of CI/CD-Integrated Linting
- Early Issue Detection: Running code linters as part of CI/CD pipelines helps detect and address code quality issues early in the development process, before changes reach production environments.
- Consistent Code Quality: By enforcing coding standards and best practices automatically, CI/CD-integrated linters promote consistency across codebases and minimize manual oversight of code reviews.
- Streamlined Code Reviews: Automated linting checks streamline code review processes by reducing the need for reviewers to focus on basic stylistic or formatting concerns, allowing them to concentrate on more substantial code improvements.
- Enhanced Collaboration: CI/CD-integrated linters encourage collaboration and knowledge sharing among team members by fostering a shared understanding of coding standards and facilitating constructive feedback loops.
Implementing Code Linters in CI/CD Pipelines
Integrating code linters into your CI/CD pipelines involves configuring automated tasks that run linters against code changes and report any identified issues. Here are some steps to implement code linters effectively within your CI/CD workflows:
- Install Code Linter: Ensure that the necessary code linter tool is installed and available within your CI/CD environment, either as a global package or a project dependency.
- Configure Linting Scripts: Set up scripts or commands in your CI/CD pipeline configuration files (e.g.,
.gitlab-ci.yml
,Jenkinsfile
) to execute linting checks against your codebase.
- Define Thresholds and Policies: Establish thresholds for acceptable code quality levels and define policies for handling linting failures, such as blocking commits or triggering alerts for severe issues.
- Run Linting Checks: Trigger linter execution during specific pipeline stages, such as code validation or automated testing, to ensure that code quality assessments are an integral part of the pipeline flow.
- Generate Reports and Feedback: Capture linting results and generate reports detailing identified issues, severity levels, and suggested fixes to provide developers with actionable feedback.
- Integrate with Version Control: Link linting reports and status indicators to your version control system (e.g., Git, SVN) to track code quality trends, monitor improvements, and facilitate visibility into linter findings.
By integrating code linters into your CI/CD pipelines and aligning them with your development processes, you can institutionalize code quality standards, automate quality assurance checks, and bolster the efficiency and reliability of your software delivery pipeline.
The Future of Code Linters: Trends and Innovations
As software development practices continue to evolve and technology landscapes shift rapidly, the realm of code linting is also experiencing advancements and innovations to meet the demands of modern development environments. In this final section, we’ll delve into emerging trends and future directions shaping the landscape of code linters.
Machine Learning-Powered Linting
The integration of machine learning and artificial intelligence technologies into code linting tools presents an exciting opportunity to enhance the detection of complex code quality issues, predict potential defects, and provide more intelligent recommendations for code improvements.
Tools leveraging machine learning algorithms can analyze vast volumes of code patterns, identify subtle anomalies, and offer tailored suggestions based on learned patterns and historical data, augmenting the capabilities of traditional rule-based linters.
Language-Agnostic Linting Solutions
With the growing popularity of polyglot development environments and the widespread adoption of multi-language codebases, there is a trend towards developing language-agnostic linting solutions that can accommodate diverse programming languages within a unified framework.
Language-agnostic linters aim to provide consistent code quality assessments, enforce generic coding standards, and offer cross-platform compatibility, eliminating the need for developers to switch between multiple linters for different languages in a project.
Real-Time Linting Integrations
Real-time code linting integrations within popular integrated development environments (IDEs) and code editors are becoming increasingly prevalent, offering immediate feedback on code quality issues as developers write and edit code.
By embedding linter functionalities directly into the coding environment, developers can receive instant notifications, suggestions, and corrections for violations of coding standards, enabling them to address issues proactively and iteratively refine their code as they work.
Enhanced Customization and Extensibility
Future code linters are expected to provide enhanced customization options and extensibility features, allowing developers to tailor linting rules, plugins, and configurations to match the specific requirements of their projects and coding styles effectively.
Advanced customization capabilities enable teams to design bespoke linting setups, enforce domain-specific guidelines, and integrate project-specific checks seamlessly, empowering them to maintain code quality standards that align precisely with their development objectives.
Cloud-Based Collaborative Linting Platforms
Cloud-based collaborative linting platforms are emerging as centralized hubs for managing code quality assessments, conducting linting analyses across distributed repositories, and fostering team-wide collaboration on code style and best practices.
These platforms offer shared linting configurations, real-time insights into code quality trends, and collaborative features for discussing and resolving linting issues collectively, promoting uniformity and coherence in coding standards across geographically dispersed teams.
By embracing these evolving trends and innovations in code linting, software development teams can adapt to changing technological landscapes, streamline code quality assurance processes, and elevate the overall quality and reliability of their codebases.
Conclusion
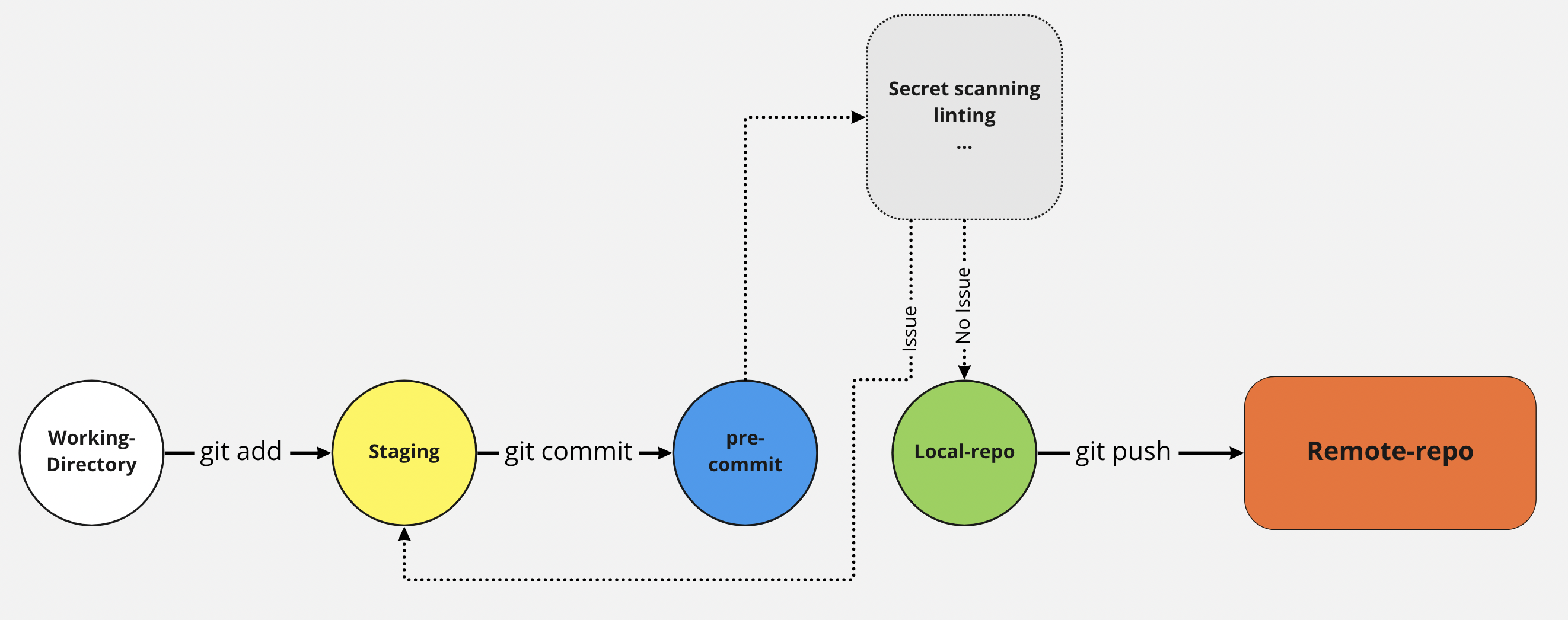
In conclusion, code linters serve as invaluable tools in the realm of software development, enabling developers to uphold code quality, enforce coding standards, and identify potential issues proactively. By integrating code linters into development workflows, setting up effective configurations, and following best practices, teams can optimize their code quality assurance processes and foster collaboration and consistency across projects.
Understanding the importance of code quality, the functioning of code linters, and the diverse range of popular linters available equips developers with the knowledge and tools necessary to elevate their coding practices and deliver high-quality software products. By addressing common issues detected by linters, integrating linting into CI/CD pipelines, and exploring future trends in code linting, developers can stay ahead of the curve and embrace innovations that enhance their coding efficiency and reliability.
As the landscape of software development continues to evolve, code linters remain essential allies in the quest for clean, maintainable codebases and efficient development practices. By staying informed about the latest developments in code linting and incorporating linters strategically into their workflows, developers can navigate the complexities of modern coding environments with confidence and precision.